Crasheye Unity Plugin
Intro
Crasheye Unity Plugin is an exception monitoring plugin exclusively for Unity game apps, which catches exceptions thrown by scripts (such as JavaScript and C#) and native code (such as Objective-C, Java) in Unity project, and offers instant and accurate error analyzing service.
Create A New App and Get The AppKey
Once you register and log in Crasheye, you can create your own app. Every app will have a unique AppKey to initialize the SDK, and to identify your app as well. ( Download Sample )
Create new app:1. Log in Crasheye to access admin panel. 2. Click the profile picture at the upper right corner of the page, and select [my apps] in the popup menu to access the list of your apps. 3. Click [new] in app list page. 4. Name your app and choose a platform in the popup box. Click [yes]. A new app is created.
Keep your unique AppKey secured from misuse.
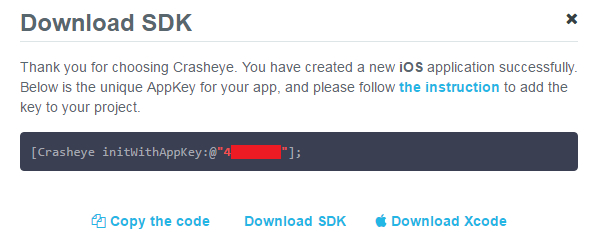
Download SDK
Click Download Unity Plugin SDK in SDK download page.
Import Crasheye Unity Plugin
In your Unity project, click on [Assets] -> [Import Package] -> [Custom Package], and select the downloaded [CrasheyeUnityPlugin.unitypackage] to import. (
Download Sample )
Select all files in importing package option box (by default), and click [Import].
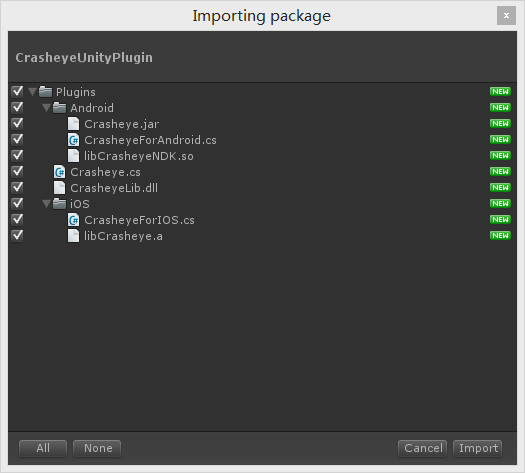
Initialize
Android MONO stack backtrace configuration
Note: Skip this step if your app will not be published on Android market.
This functionality is only for Unity 4.6.3 or later. Backtrace for Unity 4.6.3 or older will cause exceptions.
1. Export Java project:
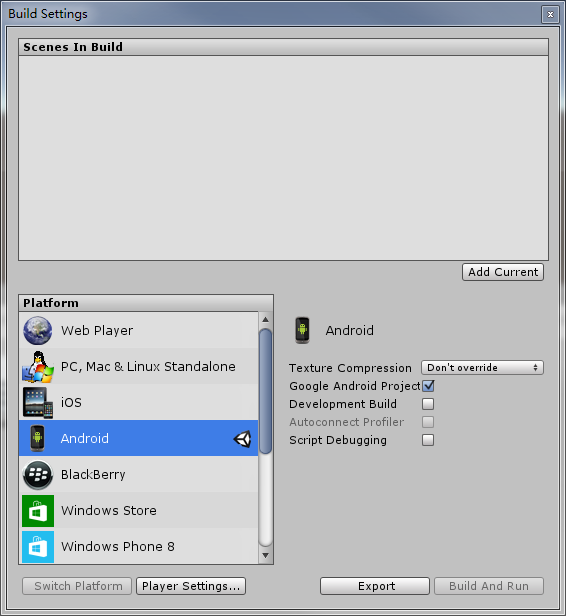
2. Initialize the exported Java project (Unity5.0: in UnityPlayerActivity.java; Unity4.6.X: in UnityPlayerNativeActivity.java)
// import header file
import com.xsj.crasheye.Crasheye;
// add initialization interface in onCreate function
Crasheye.initWithMonoNativeHandle(this, "Your_android_AppKey");

If the main Activity of your project inherits from UnityPlayerActivity, please initialize Crasheye after super.onCreate(). Because newUnityPlayer() will be called in super.onCreate(). And the new version Unity will capture exceptions. So it’s supposed to initialize Crasheye after initializing Unity so that it can make Crasheye capture exceptions firstly.

3. Export src project into a jar file:
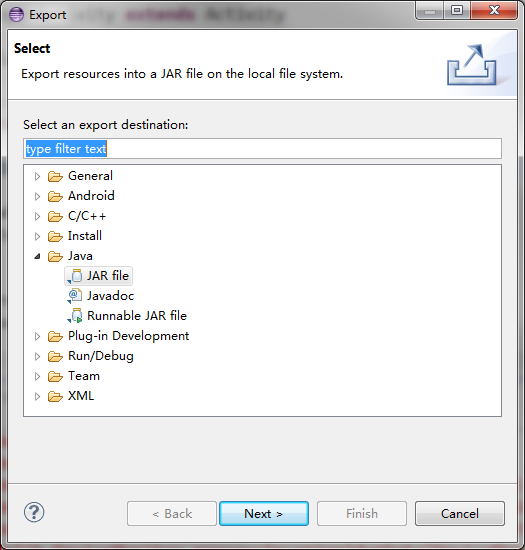
4. Deselect all files except the files in src folder, and set the exported file as MonoCrasheye.jar
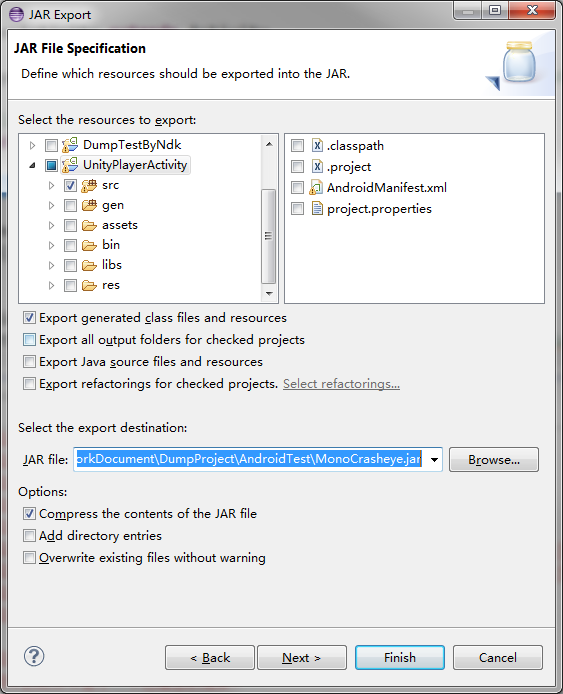
5. Add Unity init script in the entry function of project: (remove Crasheye.cs from objects before using this interface)
void Start()
{
#if UNITY_ANDROID
// Initialize the script exception catching interface in the entry function
Crasheye.RegisterLogCallback();
#endif
#if UNITY_IPHONE
// iOS initialization should be done in Unity
Crasheye.StartInitCrasheye("Your_iOS_AppKey");
#endif
}
6. Place the exported .jar file and AndroidManifest.xml in Android folder under the Unity project.
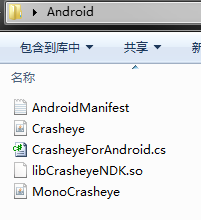
Your Android app is now configured with Unity project.
Test
Please test on a real device. If successful, data will be shown in admin panel in real time.
Run your project. If Crasheye outputs logs demonstrated below, the initialization is completed.
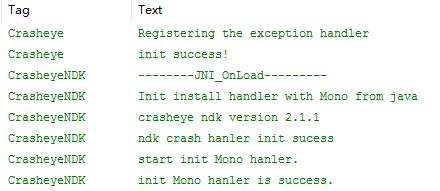
iOS App Configuration
Note: Skip this step if your app will not be published on iOS market.
Configure build settings in the iOS project exported from Unity. (Do not configure duplicate)
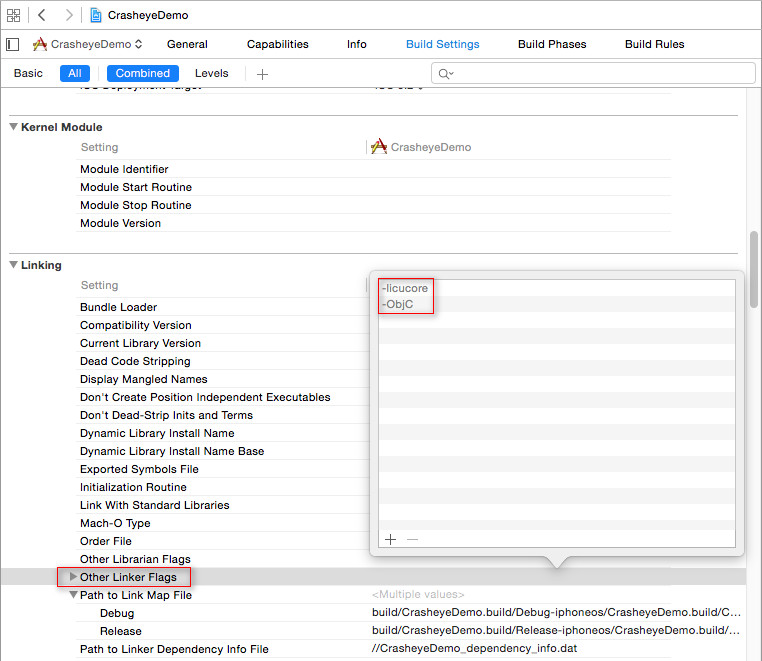
Open Xcode project settings, select [Build Phases] tab, and unfold [Link Binary with Libraries], and click [+] button:
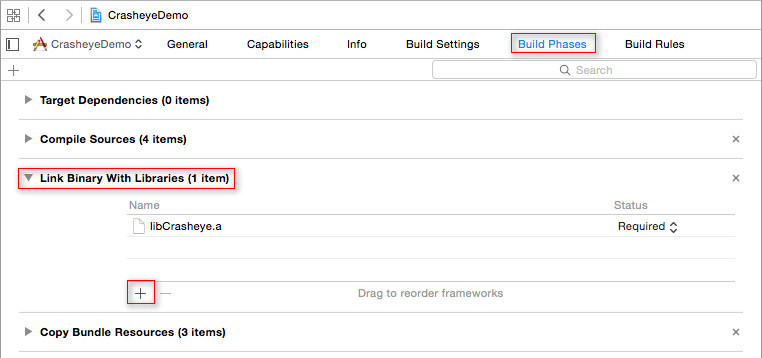
Add [libz.dylib] and [libc++.dylib] file:

Your iOS app is now configured with Unity project. Please test on a real device. If successful, data will be showed in admin panel in real time.
In iOS SDK 2.5.0 or later, it uploads files through HTTPS protocol instead, so it's not necessary to add NSExceptionDomains or NSAllowsArbitraryLoadsz fields. But in the versions older than 2.5.0, you have to add the two fields by yourself.
iOS SDK Integration in MONO Mode
Default mode for iOS SDK is IL2CPP. If MONO mode is required, you can follow the instructions below: (Skip this step if you are using IL2CPP)
1. Comment out the following code in public static void Init(string appKeyForIOS, string channIdForIOS) of CrasheyeForIOS.cs file.
crasheyeInitWithChannel(appKeyForIOS, channIdForIOS);
2. Add function declaration in main.mm of the exported Xcode project:
extern "C" void mono_set_signal_chaining(int chain_signals);
extern "C" void crasheyeInitWithChannel(const char * appkey, const char * channel);
3. Add the following code between NSAutoreleasePool* pool = [NSAutoreleasePool new]; UnityInitTrampoline(); in the main function of main.mm of the exported Xcode project.
mono_set_signal_chaining(1);
//The values of parameter "appkey" and parameter "channel" should be the real AppKey ID and Channel ID.
crasheyeInitWithChannel("appkey","channel");
Test
Please test on a real device. If successful, data will be showed in admin panel in real time.
Android Configuration for Regular Integration
Note: Skip this step if you have configured Android MONO stack backtrace.
Mount and Initialize Scripts
Select the first scene or main sceneto load in your Unity project, and choose an existing object or create a new object. Then, you need to drag [Crasheye.cs] script to the new game object to mount on it. (Sample download)
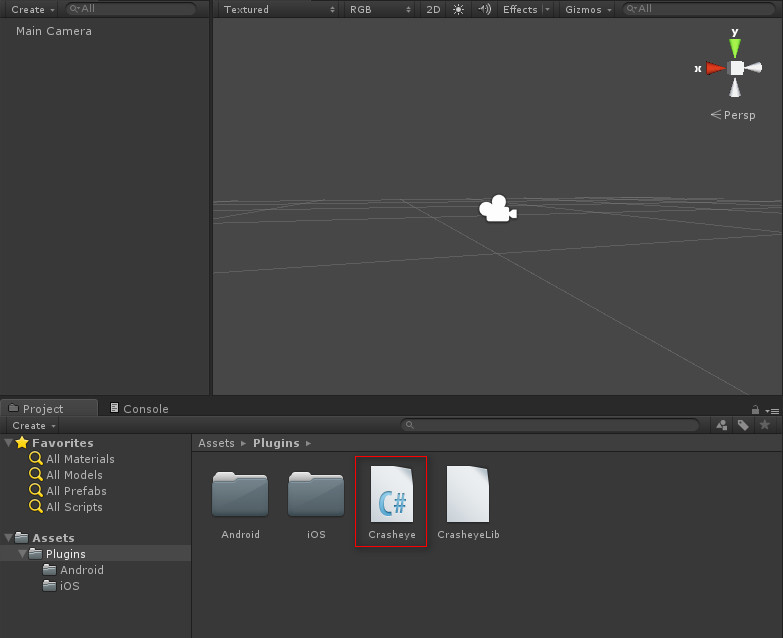
Configure AppKey
Set the Crasheye initialization in Inspector and replace the value of parameters such as Your AppKey For Android
,Your AppKey For iOS
,Your Channel Id For Android
,Your Channel Id For iOS
with the corresponding AppKey and Channel ID. AppKey is mandatory. Channel ID can be optional.
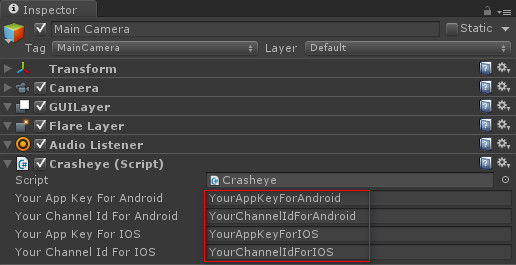
Open [Build Setting] panel. Select an Android platform, and click on [Player Settings...]. Then, you can switch to [Setting for Android] tab to unfold [Other Settings], and select [Require] in [Internet Access].
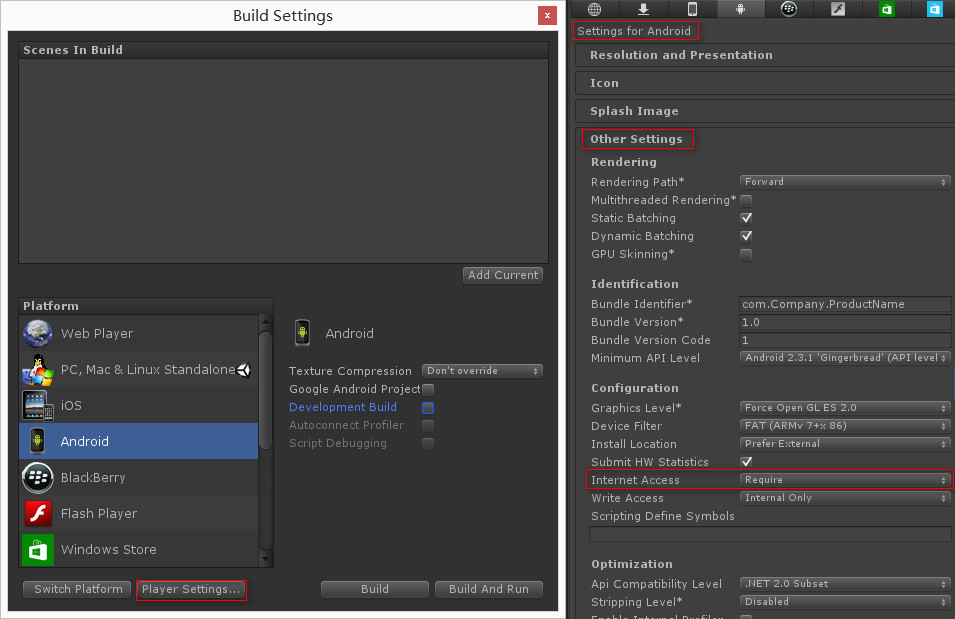
Finally, please make sure that Android permission permission has been included in [AndroidManifest.xml] of the exported Android project.
Note: Do not configure duplicate.
Note: Do not configure duplicate.
Your Android app is now configured with Unity project. Please test on a real device. If successful, real time data will be monitored and shown on the Crasheye admin panel.
Common APIs
Report Script Exception
This API is for the convenience of developers. The caught exceptions of stack trace, such as lua and js script exceptionexceptions of stack trace, will be reported to server.
/**
* Script exception reporting interface
* @param e : exception information
*/
Crasheye.sendScriptException(Exception e);
Upload via Wifi Only
In order to save the cellular data usage for the users, Crasheye offers an API to report stack trace of crashes only when the device is on wifi networks.
/**
* Whether the file is uploaded via WIFI only or not
* @param enabled true: Report via WIFI only
*/
Crasheye.setFlushOnlyOverWiFi(bool enabled);
App Version
By default, Crasheye will read version information from configuration file. Of course you can also set app version by calling API .
Crasheye.SetAppVersion(string youAppVersion);
User Identification
You can assign User Identification to each reported issue to help filter and locate issues. Developer can use this method to filter crash issues reported by his own device.
/**
* Set user identifier
* @param userIdentifier : user identifier
*/
Crasheye.SetUserIdentifier("UserIdentifiter");
Channel ID
You can call an API to set Channel ID:
/**
* Set channel ID
* @param ChannelID Set channel ID
*/
Crasheye.SetChannelID("xxx");
Developer-Defined Text String
In case that you want to capture more data than the default setup does, you can call this API to add developer-defined text string to collect more needed information:
/**
* Add User-defined keyword
* @param key : user-defined identifier
* @param value : user-defined information
*/
Crasheye.AddExtraData(string key, string value);
Breadcrumb (Navigation Information)
By leaving breadcrumbs (navigation information), you can capture the time and sequence of crashes and handled exceptions to keep track of if your app is working as intended. Call the following API where you want to monitor:
Crasheye.leaveBreadcrumb(string breadcrumb);
Log Collection
This API collects logs (LogCat) in runtime. When exception occurs, the collected logs will be saved and reported to server. (Maximum 1000 lines of the latest logs)
The interface is for Android system only, and is not working in iOS system.
/**
* Access application log (filtration parameters: keyword and line count)
* @param lines : lines of codes collected (1000 lines in maximum)
* @param filter : Keyword(require = false), for example, using Crasheye as keyword is equivalent to entering "logcat -d Crasheye" in Logcat filter
*/
Crasheye.SetLogging(lines, filter);
or
Crasheye.SetLogging(lines);
Register Log Call Back Function
Application.RegisterLogCallback is the function that calls back register log. This interface is initialized only once in Crasheye, so you can set the function you need using this interface if necessary.
/**
* configure the function that calls back register log
* @param RegisterLog the function that needs to be called back
*/
Crasheye.SetRegisterLogFunction(RegisterLog);
//eq:
void Start()
{
Crasheye.SetRegisterLogFunction(Test1);
}
[AOT.MonoPInvokeCallback(typeof(Crasheye.RegisterLog))]
public void RegisterLogFunction(string logString, string stackTrace, LogType type)
{
//do something
}
Set Beta Version
/**
* Set whether the version is the beta
* @param isBeta : true is beta version(true or false)
*/
Crasheye.SetIsBetaVersion(isBeta)