Crasheye SDK for iOS
Create A New App and Get The AppKey
Once you register and log in Crasheye, you can create your own app. Every app will have a unique AppKey to initialize the SDK, and to identify your app as well.
Create new app:1. Log in Crasheye to access admin panel. 2. Click the profile picture at the upper right corner of the page, and select [my apps] in the popup menu to access the list of your apps. 3. Click [new] in app list page. 4. Name your app and choose a platform in the popup box. Click [yes]. A new app is created.
Keep your unique AppKey secured from misuse.
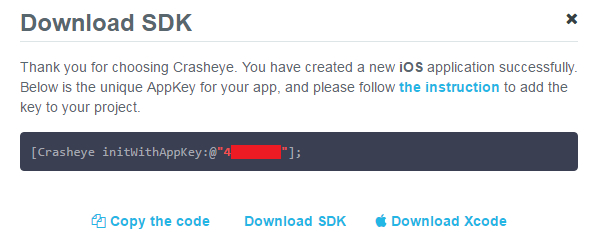
Download SDK
Click Download iOS SDK in SDK download page.
Import iOS SDK
Drag the downloaded [Crasheye.h] and [libCrasheye.a] into your Xcode project as shown:
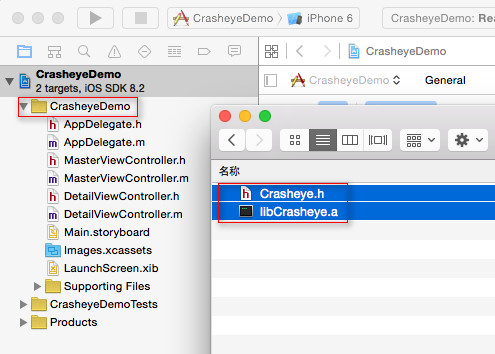
You can also right click on your Xcode project, and select [Add Files to "xxxx"...]:
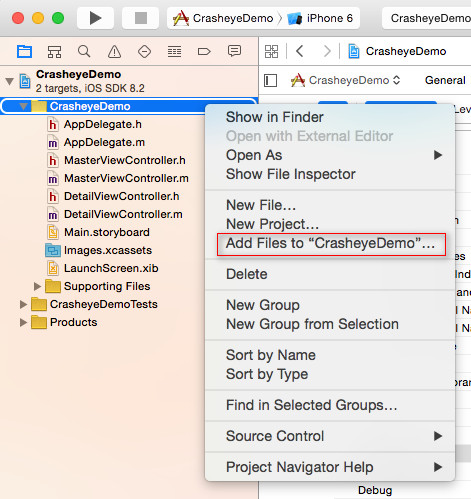
Select [Copy items if needed] in the pop-up box and select your targets in [Add To Targets].
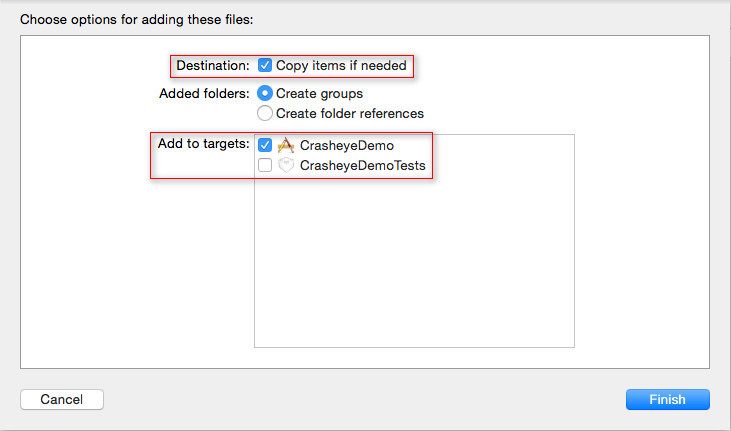
Configuration
Open Xcode project settings, select [Build Phases] tab, unfold [Link Binary with Libraries], and click [+] button:
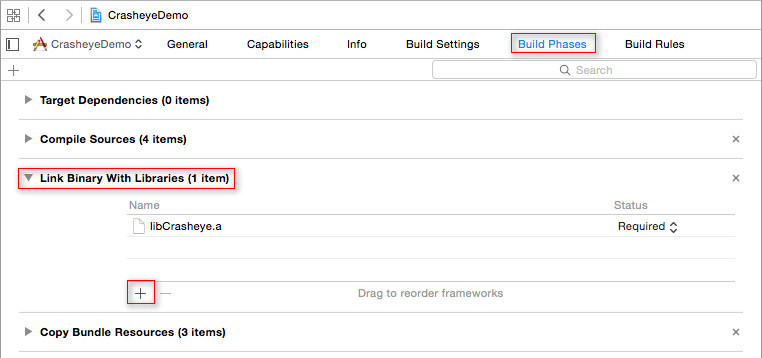
Add [libz.dylib] and [libc++.dylib] file:

Configure build settings for your project:
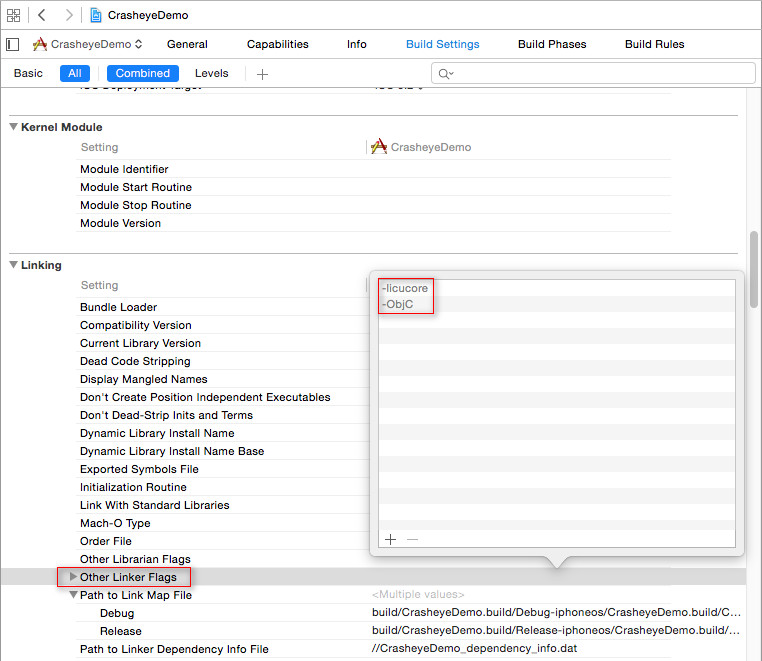
In iOS SDK 2.5.0 or later, it uploads files through HTTPS protocol instead, so it's not necessary to add NSExceptionDomains or NSAllowsArbitraryLoadsz fields. But in the versions older than 2.5.0, you have to add the two fields by yourself.
Initialize SDK
Import header file in the source file [AppDelegate.m] of the project
#import "Crasheye.h"
Initialize SDK by using application:didFinishLaunchingWithOptions:
as following:
[Crasheye initWithAppKey:@"Your_AppKey"]
Initialize SDK as following if you want to specify Channel ID of your app:
[Crasheye initWithAppKey:@"Your_AppKey" withChannel:@"Your_Channel"]
Your iOS app is now integrated with Crasheye. Start the app on your Apple device, and real time data will be monitored and shown on the Crasheye admin panel.
Test
Please test on a real device. If successful, data will be shown in admin panel in real time.
Start your application. Real time data will be monitored and shown on the Crasheye admin panel instantly.
Common APIs
Set Version No.
Available to set version number by yourself if necessary, and also the SDK gets app's version information by default. Note: Must be called before Crasheye init.
[Crasheye setAppVersion:(NSString *) appVersion];
User Identification
You can assign User Identification to each reported issue to help filter and locate issues. Developer can use this method to filter crash issues reported by his own device.
[Crasheye setUserID:(NSString *) userID];
Breadcrumb (Navigation Information)
By leaving breadcrumbs (navigation information), you can capture the time and sequence of crashes and handled exceptions to keep track of if your app is working as intended. Call the following API where you want to monitor:
[Crasheye leaveBreadcrumb:(NSString *) breadcrumb];
Developer-Defined Text String
In case that you want to capture more data than the default setup does, you can call this API to add developer-defined text string to collect more information:
[Crasheye addExtraDataWithDic:(NSDictionary *) dic];
Report Script Exception
This API is for the convenience of developers. The caught exceptions of stack trace, such as lua and js script exception, will be reported to serverexceptions of stack trace. (In order to reduce repetitive reports, the same exception will only be reported once in 10 minutes)
/*
@param:
errorTitle errorTile: title of script exception, specified by developer. It cannot be null.
exception exception: description of exception. It cannot null.
*/
[Crasheye sendScriptExceptionRequestWithTitle:(NSString *) errorTitle exception:(NSString *) exception file:(NSString *) file language:(NSString *) language];
Device ID
Device ID is accessible via this API. Users can filter the logs with device ID to find the corresponding exceptions of the device.
[Crasheye getDeviceID];
Set Beta Version
/**
* Set whether the version is the beta
* @param enabled : 1 is beta version, 0 is not
*/
[Crasheye setBeta:(int32_t) enabled];
Symbol File Upload
1. Environment
Java SE Runtime Environment 7 or later
2. Preparation
a. Symbol file upload tool
b. dSYM file (containing debugging information in iOS system). Location: Xcode -> project -> product -> xxx.app -> click on [show file location] -> xxx.app.dSYM
Xcode -> project -> product -> xxx.app -> click on [show file location] -> xxx.app.dSYM
3. Upload Symbol File
Arguments:-appkey appkey for the project
[-veresion] app version(optional), read from Contents/info.plist in symbol file by default
[-platform] Default value is iOS.
[-disableUpload] disable symbol file upload
[-dumpsyms] This is the location of dump_syms, which is a dsym analyzing system. It points to the current working directory by default.
Symbol file path
Via Website:
1. Start the symbol file upload tool, and use the following command to upload:
java -jar CrasheyeiOSSymbol.jar -disableUpload symbol file path
2. Upload the compressed file (.zip file) via website (Settings -> Symbols -> Symbol File Upload), and select the corresponding app version.
Via Tool:
3. Start the symbol file upload tool, and use the following command to upload:
java -jar CrasheyeiOSSymbol.jar -appkey=project's AppKey [-version=App version] [-platform=ios] symbol_file_path